문제 1 |
배달음식 전문점 운영을 위해 다음과 같이 DeliveryStore 추상 클래스와 PizzaStore, Food 클래스를 작성했습니다.
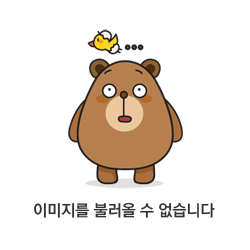
* DeliveryStore :
* DeliveryStore는 배달 음식점의 추상 클래스 입니다.
* 배달 음식점은 set_order_list와 get_total_price 함수를 구현해야 합니다.
* set_order_list 함수는 주문 메뉴의 리스트를 매개변수로 받아 저장합니다.
* get_total_price 함수는 주문받은 음식 가격의 총합을 return 합니다.
* Food :
* Food는 음식을 나타내는 클래스입니다.
* 음식은 이름(name)과 가격(price)으로 구성되어있습니다.
* PizzaStore
* PizzaStore는 피자 배달 전문점을 나타내는 클래스이며 추상 클래스인 DeliveryStore의 파생 클래스입니다.
* menu_list는 피자 배달 전문점에서 주문 할 수 있는 음식의 리스트를 저장합니다.
* order_list는 주문 받은 음식들의 이름을 저장합니다.
* set_order_list 함수는 주문 메뉴를 받아 order_list에 저장합니다.
* get_total_price 함수는 order_list에 들어있는 음식 가격의 총합을 return 합니다.
주문 메뉴가 들어있는 배열 order_list가 매개변수로 주어질 때, 주문한 메뉴의 전체 가격을 return 하도록 solution 함수를 작성하려고 합니다. 위의 클래스 구조를 참고하여 주어진 코드의 빈칸을 적절히 채워 전체 코드를 완성해주세요.
---
##### 매개변수 설명
주문 메뉴가 들어있는 배열 order_list가 solution 함수의 매개변수로 주어집니다.
* order_list의 길이는 1 이상 5이하입니댜.
* order_list에는 주문하려는 메뉴의 이름들이 문자열 형태로 들어있습니다.
* order_list에는 같은 메뉴의 이름이 중복해서 들어있지 않습니다.
* 메뉴의 이름과 가격은 PizzaStore의 생성자에서 초기화해줍니다.
---
##### return 값 설명
주문한 메뉴의 전체 가격을 return 해주세요.
##### 예시
order_list | return |
["Cheese", "Pineapple", "Meatball"] | 51600 |
[ 소스 코드 ]
#include <iostream>
#include <cstdlib>
#include <vector>
#include <string>
using namespace std;
class DeliveryStore{
public:
virtual void set_order_list(vector<string> order_list) = 0;
virtual int get_total_price() = 0;
};
class Food{
public:
string name;
int price;
Food(string name, int price){
this->name = name;
this->price = price;
}
};
class PizzaStore : @@@{
private:
vector<Food> menu_list;
vector<string> order_list;
public:
PizzaStore(){
//init menu_list
menu_list = {{"Cheese", 11100}, {"Potato", 12600}, {"Shrimp", 13300},{"Pineapple", 21000}, {"Meatball", 19500}};
//init order_list
order_list.clear();
}
@@@{
for(int i = 0; i < order_list.size(); i++)
this->order_list.push_back(order_list[i]);
};
@@@{
int total_price = 0;
for(int i = 0; i < order_list.size(); i++){
for(int j = 0; j < menu_list.size(); j++)
if(order_list[i] == menu_list[j].name){
total_price += menu_list[j].price;
break;
}
}
return total_price ;
};
};
int solution(vector<string> order_list){
DeliveryStore* delivery_store = new PizzaStore();
delivery_store->set_order_list(order_list);
int total_price = delivery_store->get_total_price();
return total_price;
}
// The following is main function to output testcase.
int main() {
vector<string> order = {"Cheese", "Pineapple", "Meatball"};
int ret = solution(order);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret << " ." << endl;
return 0;
}
[ 정답보기 ]
#include <iostream>
#include <cstdlib>
#include <vector>
#include <string>
using namespace std;
class Food{
public:
string name;
int price;
Food(string name, int price){
this->name = name;
this->price = price;
}
};
class DeliveryStore{
public:
virtual void set_order_list(vector<string> order_list) = 0;
virtual int get_total_price() = 0;
};
class PizzaStore : public DeliveryStore{
private:
vector<Food> menu_list;
vector<string> order_list;
public:
PizzaStore(){
//init menu_list
menu_list = {{"Cheese", 11100}, {"Potato", 12600}, {"Shrimp", 13300},{"Pineapple", 21000}, {"Meatball", 19500}};
//init order_list
order_list.clear();
}
void set_order_list(vector<string> order_list){
for(int i = 0; i < order_list.size(); ++i)
this->order_list.push_back(order_list[i]);
};
int get_total_price(){
int total_price = 0;
for(int i = 0; i < order_list.size(); ++i){
for(int j = 0; j < menu_list.size(); ++j)
if(order_list[i] == menu_list[j].name){
total_price += menu_list[j].price;
break;
}
}
return total_price ;
};
};
int solution(vector<string> order_list){
DeliveryStore* delivery_store = new PizzaStore();
delivery_store->set_order_list(order_list);
int total_price = delivery_store->get_total_price();
return total_price;
}
문제 2 |
해밍 거리(Hamming distance)란 같은 길이를 가진 두 개의 문자열에서 같은 위치에 있지만 서로 다른 문자의 개수를 뜻합니다. 예를 들어 두 2진수 문자열이 "10010"과 "110"이라면, 먼저 두 문자열의 자릿수를 맞추기 위해 "110"의 앞에 0 두개를 채워 "00110"으로 만들어 줍니다. 두 2진수 문자열은 첫 번째와 세 번째 문자가 서로 다르므로 해밍 거리는 2입니다.
* `1`0`0`1 0
* `0`0`1`1 0
두 2진수 문자열 binaryA, binaryB의 해밍 거리를 구하려 합니다. 이를 위해 다음과 같이 간단히 프로그램 구조를 작성했습니다
~~~
1단계. 길이가 더 긴 2진수 문자열의 길이를 구합니다.
2단계. 첫 번째 2진수 문자열의 길이가 더 짧다면 문자열의 앞에 0을 채워넣어 길이를 맞춰줍니다.
3단계. 두 번째 2진수 문자열의 길이가 더 짧다면 문자열의 앞에 0을 채워넣어 길이를 맞춰줍니다.
4단계. 길이가 같은 두 2진수 문자열의 해밍 거리를 구합니다.
~~~
두 2진수 문자열 binaryA와 binaryB가 매개변수로 주어질 때, 두 2진수의 해밍 거리를 return 하도록 solution 함수를 작성했습니다. 이때, 위 구조를 참고하여 중복되는 부분은 func_a라는 함수로 작성했습니다. 코드가 올바르게 동작할 수 있도록 빈칸을 알맞게 채워 전체 코드를 완성해주세요.
---
##### 매개변수 설명
두 2진수 문자열 binaryA와 binaryB가 solution 함수의 매개변수로 주어집니다.
* binaryA의 길이는 1 이상 10 이하입니다.
* binaryA는 0 또는 1로만 이루어진 문자열이며, 0으로 시작하지 않습니다.
* binaryB의 길이는 1 이상 10 이하입니다.
* binaryB는 0 또는 1로만 이루어진 문자열이며, 0으로 시작하지 않습니다.
---
##### return 값 설명
두 2진수 문자열의 해밍 거리를 return 해주세요.
---
##### 예시
binaryA | binaryB | return |
"10010" | "110" | 2 |
##### 예시 설명
두 2진수의 자릿수는 각각 5와 3입니다. 자릿수를 맞추기 위해 "110" 앞에 0 두 개를 채워주면 "00110"이 됩니다. 이제 두 2진수 문자열의 해밍 거리를 구하면 다음과 같습니다.
* `1`0`0`1 0
* `0`0`1`1 0
위와 같이 첫 번째와 세 번째 문자가 서로 다르므로, 해밍 거리는 2가 됩니다.
[ 소스 코드 ]
#include <iostream>
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
string func_a(string str, int len){
string padZero = "";
int padSize = @@@;
for(int i = 0; i < padSize; i++)
padZero += "0";
return padZero + str;
}
int solution(string binaryA, string binaryB) {
int max_length = max(binaryA.length(), binaryB.length());
binaryA = func_a(binaryA, max_length);
binaryB = func_a(binaryB, max_length);
int hamming_distance = 0;
for(int i = 0; i < max_length; i++)
if(@@@)
hamming_distance += 1;
return hamming_distance;
}
// The following is main function to output testcase.
int main() {
string binaryA = "10010";
string binaryB = "110";
int ret = solution(binaryA, binaryB);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret << " ." << endl;
}
[ 정답 보기 ]
#include <string>
#include <vector>
#include <algorithm>
using namespace std;
string func_a(string str, int len){
string padZero = "";
int padSize = len - str.length();
for(int i = 0; i < padSize; ++i)
padZero += "0";
return padZero + str;
}
int solution(string binaryA, string binaryB) {
int max_length = max(binaryA.length(), binaryB.length());
if(max_length > binaryA.length())
binaryA = func_a(binaryA, max_length);
if(max_length > binaryB.length())
binaryB = func_a(binaryB, max_length);
int hamming_distance = 0;
for(int i = 0; i < max_length; ++i)
if(binaryA[i] != binaryB[i])
hamming_distance += 1;
return hamming_distance;
}
문제 3 |
문자열 형태의 식을 계산하려 합니다. 식은 2개의 자연수와 1개의 연산자('+', '-', '*' 중 하나)로 이루어져 있습니다. 예를 들어 주어진 식이 "123+12"라면 이를 계산한 결과는 135입니다.
문자열로 이루어진 식을 계산하기 위해 다음과 같이 간단히 프로그램 구조를 작성했습니다.
~~~
1단계. 주어진 식에서 연산자의 위치를 찾습니다.
2단계. 연산자의 앞과 뒤에 있는 문자열을 각각 숫자로 변환합니다.
3단계. 주어진 연산자에 맞게 연산을 수행합니다.
~~~
문자열 형태의 식 expression이 매개변수로 주어질 때, 식을 계산한 결과를 return 하도록 solution 함수를 작성하려 합니다. 위 구조를 참고하여 코드가 올바르게 동작할 수 있도록 빈칸에 주어진 func_a, func_b, func_c 함수와 매개변수를 알맞게 채워주세요.
---
##### 매개변수 설명
문자열 형태의 식 expression이 solution 함수의 매개변수로 주어집니다.
* expression은 연산자 1개와 숫자 2개가 결합한 형태입니다.
* 연산자는 '+', '-', '*'만 사용됩니다.
* 숫자는 1 이상 10,000 이하의 자연수입니다.
---
##### return 값 설명
expression을 계산한 결과를 return 해주세요.
* 계산 결과는 문자열로 변환하지 않아도 됩니다.
---
##### 예시
expression | return |
"123+12" | 135 |
##### 예시 설명
'+'를 기준으로 앞의 숫자는 123이고 뒤의 숫자는 12이므로 두 숫자를 더하면 135가 됩니다.
[ 소스 코드 ]
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Pair{
public:
int first_num;
int second_num;
};
int func_a(int numA, int numB, char exp){
if (exp == '+')
return numA + numB;
else if (exp == '-')
return numA - numB;
else
return numA * numB;
}
int func_b(string exp){
for(int i = 0; i < exp.length(); i++){
char e = exp[i];
if(e == '+' || e == '-' || e == '*')
return i;
}
return -1;
}
Pair func_c(string exp, int idx){
Pair ret;
ret.first_num = stoi(exp.substr(0, idx));
ret.second_num = stoi(exp.substr(idx+1));
return ret;
}
int solution(string expression) {
int exp_index = func_@@@(@@@);
Pair numbers = func_@@@(@@@);
int result = func_@@@(@@@);
return result;
}
// The following is main function to output testcase.
int main() {
string expression = "123+12";
int ret = solution(expression);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret << " ." << endl;
}
[ 정답 보기 ]
#include <string>
#include <vector>
using namespace std;
class Pair{
public:
int first_num;
int second_num;
};
int func_a(int numA, int numB, char exp){
if (exp == '+')
return numA + numB;
else if (exp == '-')
return numA - numB;
else
return numA * numB;
}
int func_b(string exp){
for(int i = 0; i < exp.length(); ++i){
char e = exp[i];
if(e == '+' || e == '-' || e == '*')
return i;
}
return -1;
}
Pair func_c(string exp, int idx){
Pair ret;
ret.first_num = stoi(exp.substr(0, idx));
ret.second_num = stoi(exp.substr(idx+1));
return ret;
}
int solution(string expression) {
int exp_index = func_b(expression);
Pair numbers = func_c(expression, exp_index);
int result = func_a(numbers.first_num, numbers.second_num, expression[exp_index]);
return result;
}
문제 4 |
어느 누군가가 타임머신을 타고 과거로 가서 숫자 0이 없는 수 체계를 전파했습니다. 역사가 바뀌어 이제 사람들의 의식 속엔 0이란 숫자가 사라졌습니다. 따라서, 현재의 수 체계는 1, 2, 3, ..., 8, 9, 11, 12, ...와 같이 0이 없게 바뀌었습니다.
0을 포함하지 않은 자연수 num이 매개변수로 주어질 때, 이 수에 1을 더한 수를 return 하도록 solution 함수를 완성해주세요.
---
#####매개변수 설명
자연수 num이 solution 함수의 매개변수로 주어집니다.
* num은 1 이상 999,999,999,999,999,999 이하의 0을 포함하지 않는 자연수입니다.
---
#####return 값 설명
자연수 num에 1을 더한 수를 return 해주세요.
---
#####예시
num | return |
9949999 | 9951111 |
#####예시 설명
9,949,999에 1을 더하면 9,950,000이지만 0은 존재하지 않으므로 9,951,111이 됩니다.
[ 소스 코드 ]
// You may use include as below.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
long long solution(long long num) {
// Write code here.
long long answer = 0;
return answer;
}
// The following is main function to output testcase.
int main() {
long long num = 9949999;
long long ret = solution(num);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret << " ." << endl;
}
[ 정답 보기 ]
// <undefined>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
long long solution(long long num) {
num++;
long long digit = 1;
while (num / digit % 10 == 0) {
num += digit;
digit *= 10;
}
return num;
}
문제 5 |
다음과 같이 n x n 크기의 격자에 1부터 n x n까지의 수가 하나씩 있습니다.
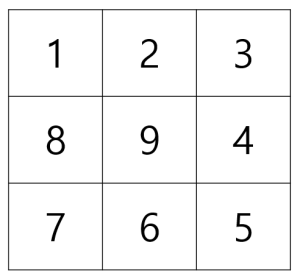
이때 수가 다음과 같은 순서로 배치되어있다면 이것을 n-소용돌이 수라고 부릅니다.
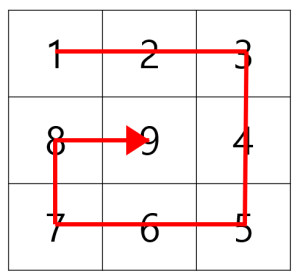
소용돌이 수에서 1행 1열부터 n 행 n 열까지 대각선상에 존재하는 수들의 합을 구해야 합니다.
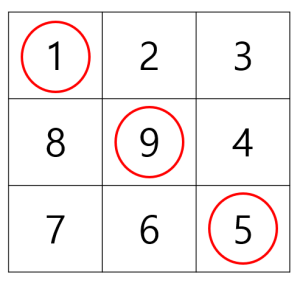
위의 예에서 대각선상에 존재하는 수의 합은 15입니다.
격자의 크기 n이 주어질 때 n-소용돌이 수의 대각선상에 존재하는 수들의 합을 return 하도록 solution 함수를 완성해주세요.
---
##### 매개변수 설명
격자의 크기 n이 solution 함수의 매개변수로 주어집니다.
* n은 1 이상 100 이하의 자연수입니다.
---
##### return 값 설명
n-소용돌이 수의 대각선상에 존재하는 수들의 합을 return 해주세요.
---
##### 예시
n | return |
3 | 15 |
2 | 4 |
[ 소스 코드 ]
// You may use include as below.
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int solution(int n) {
// Write code here.
int answer = 0;
return answer;
}
// The following is main function to output testcase.
int main() {
int n1 = 3;
int ret1 = solution(n1);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret1 << " ." << endl;
int n2 = 2;
int ret2 = solution(n2);
// Press Run button to receive output.
cout << "Solution: return value of the function is " << ret2 << " ." << endl;
}
[ 정답 코드 ]
#include <bits/stdc++.h>
#define pb push_back
#define sz(v) (int)(v).size()
#define all(v) (v).begin(),(v).end()
#define fastio() ios::sync_with_stdio(0),cin.tie(0);
using namespace std;
typedef long long ll;
const int mod = 1e9 + 7;
const int inf = 0x3c3c3c3c;
const ll infl = 0x3c3c3c3c3c3c3c3c;
int pane[103][103];
int inRange(int i, int j, int n){
return 0 <= i && i < n && 0 <= j && j < n;
}
int dy[4] = {0, 1, 0, -1};
int dx[4] = {1, 0, -1, 0};
int solution(int n){
int ci = 0, cj = 0;
int num = 1;
while(inRange(ci, cj, n) && pane[ci][cj] == 0){
for(int k = 0; k < 4; k++){
if(!inRange(ci, cj, n) || pane[ci][cj] != 0) break;
while(1){
pane[ci][cj] = num++;
int ni = ci + dy[k];
int nj = cj + dx[k];
if(!inRange(ni, nj, n) || pane[ni][nj] != 0){
ci += dy[(k + 1) % 4];
cj += dx[(k + 1) % 4];
break;
}
ci = ni;
cj = nj;
}
}
}
int ans = 0;
for(int i = 0; i < n; i++) ans += pane[i][i];
return ans;
}
'COS Pro C++ 1급 모의고사' 카테고리의 다른 글
2. COS Pro C++ 1급 모의고사 1차(6~10번) (0) | 2024.05.27 |
---|